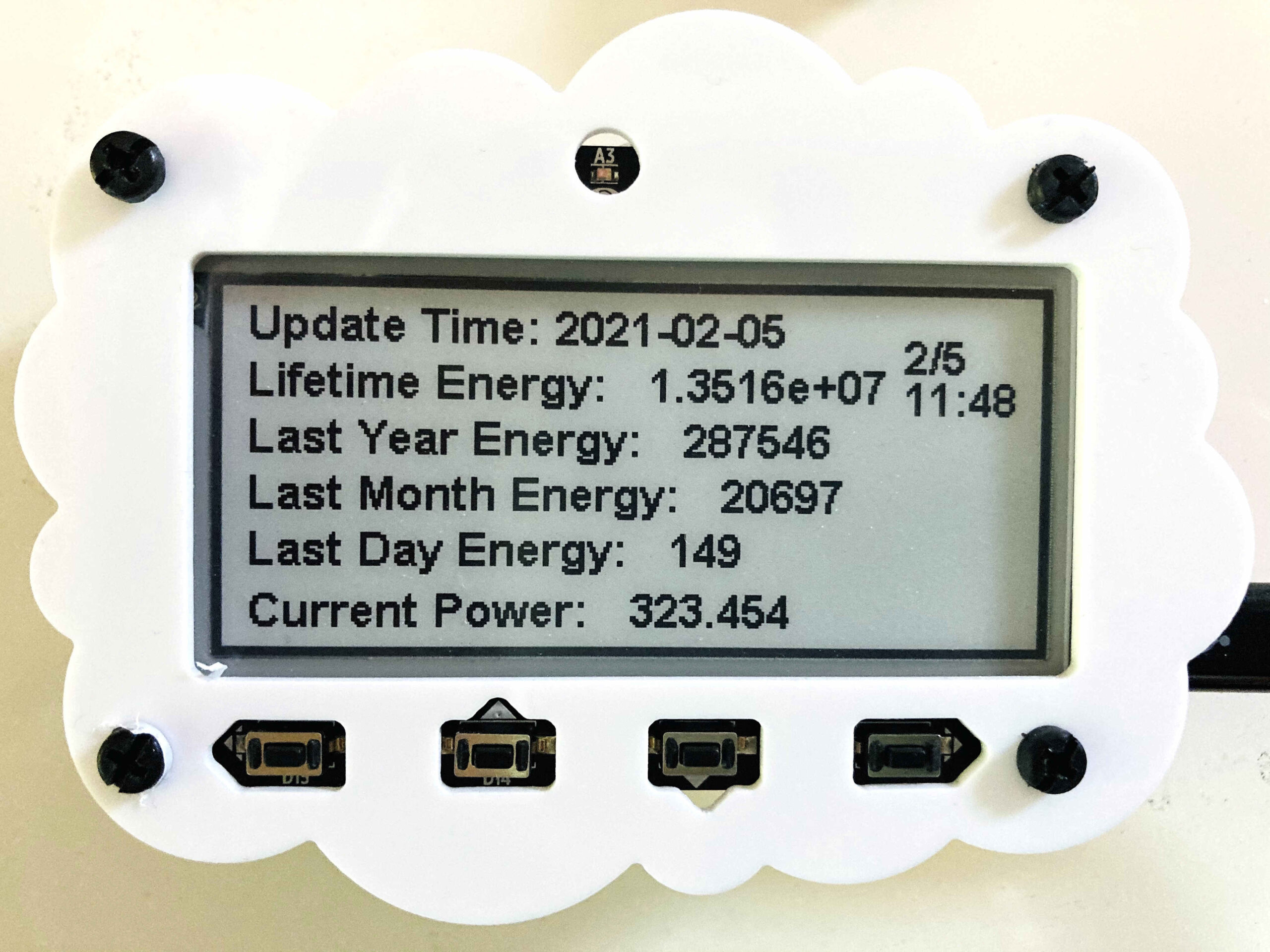
As a subscriber of the Adafruit ADABOX, I knew when I saw the low power E-Ink “MagTag” display that it would be a great way to keep track of how our solar panels are performing. In 2019 our solar panels lost a month or so of contributing to our electric supply because of a faulty inverter that we failed to notice. Ever since I’ve wanted some sort of monitor inside my house that I can keep an eye on.
I replaced Adafruit’s Covid Tracker project almost word for word, variable for variable, with the json output of the SolarEdge Dashboard api. It works like a charm, every day at the time of maximum sun, 2pm, the MagTag updates the display with data it acquires over the Wi-Fi network in my home.
The Adabox supplied the LiPo battery, the cloud cover plate, and many project ideas at their learn.adafruit.com site. Note: I did try adding an optimistic Covid-19 “percent of the nation vaccinated” data point but I couldn’t find any data sources online that can be queried with JSON.
The Circuit-Python code as snarfed and updated for SolarEdge tracking.:
# SPDX-FileCopyrightText: 2017 Scott Shawcroft, written for Adafruit Industries
#
# SPDX-License-Identifier: Unlicense
import sys
import time
import board
import digitalio
import neopixel
from adafruit_magtag.magtag import MagTag
try:
from secrets import secrets
except ImportError:
print("WiFi secrets are kept in secrets.py, please add them there!")
raise
DAILY_UPDATE_HOUR = 14
SOLAREDGE_SOURCE = "https://monitoringapi.solaredge.com/site/"+secrets['solaredge_SiteID']+"/overview?format=json&api_key="+secrets['solaredge_apikey']
LAST_UPDATE_TIME = ['overview', 'lastUpdateTime']
LIFE_TIME_DATA = ['overview', 'lifeTimeData', 'energy']
LAST_YEAR_DATA = ['overview', 'lastYearData', 'energy']
LAST_MONTH_DATA = ['overview', 'lastMonthData', 'energy']
LAST_DAY_DATA = ['overview', 'lastDayData', 'energy']
CURRENT_POWER = ['overview', 'currentPower', 'power']
magtag = MagTag(
url=SOLAREDGE_SOURCE,
json_path=(LAST_UPDATE_TIME, LIFE_TIME_DATA,
LAST_YEAR_DATA, LAST_MONTH_DATA,
LAST_DAY_DATA, CURRENT_POWER),
)
# Date stamp of info
magtag.add_text(
text_font="/fonts/Arial-Bold-12.pcf",
text_position=(10, 15),
text_transform=lambda x: "Update Time: {}".format(x[0:10]),
)
# Positive increase
magtag.add_text(
text_font="/fonts/Arial-Bold-12.pcf",
text_position=(10, 35),
text_transform=lambda x: "Lifetime Energy: {:,}".format(x),
)
# Curr hospitalized
magtag.add_text(
text_font="/fonts/Arial-Bold-12.pcf",
text_position=(10, 55),
text_transform=lambda x: "Last Year Energy: {:,}".format(x),
)
# Change in hospitalized
magtag.add_text(
text_font="/fonts/Arial-Bold-12.pcf",
text_position=(10, 75),
text_transform=lambda x: "Last Month Energy: {:,}".format(x),
)
# All deaths
magtag.add_text(
text_font="/fonts/Arial-Bold-12.pcf",
text_position=(10, 95),
text_transform=lambda x: "Last Day Energy: {:,}".format(x),
)
# new deaths
magtag.add_text(
text_font="/fonts/Arial-Bold-12.pcf",
text_position=(10, 115),
text_transform=lambda x: "Current Power: {:,}".format(x),
)
# updated time
magtag.add_text(
text_font="/fonts/Arial-Bold-12.pcf",
text_position=(245, 30),
line_spacing=0.75,
is_data=False
)
magtag.peripherals.neopixels.brightness = 0.1
magtag.peripherals.neopixel_disable = False # turn on lights
magtag.peripherals.neopixels.fill(0x0F0000) # red!
magtag.get_local_time()
try:
now = time.localtime()
print("Now: ", now)
# display the current time since its the last-update
updated_at = "%d/%d\n%d:%02d" % now[1:5]
magtag.set_text(updated_at, 6, False)
magtag.peripherals.neopixels.fill(0x0F0000) # red!
# get data from the JSON QUERY
value = magtag.fetch()
print("Response is", value)
magtag.peripherals.neopixels.fill(0x000000) #
# OK we're done!
magtag.peripherals.neopixels.fill(0x000F00)
except (ValueError, RuntimeError) as e:
print("Some error occured, trying again later -", e)
time.sleep(2) # let screen finish updating
event_time = time.struct_time((now[0], now[1], now[2],
DAILY_UPDATE_HOUR, 0, 0,
-1, -1, now[8]))
print("event_time :", event_time)
# how long is that from now?
remaining = time.mktime(event_time) - time.mktime(now)
if remaining < 0: # ah its aready happened today...
remaining += 24 * 60 * 60 # wrap around to the next day
remaining_hrs = remaining // 3660
remaining_min = (remaining % 3600) // 60
print("Gonna zzz for %d hours, %d minutes" % (remaining_hrs, remaining_min))
# Turn it all off and go to bed till the next update time
magtag.exit_and_deep_sleep(remaining)
print("waking up")
